AIM: To use Python and the Cantera library to understand the factors affecting the adiabatic flame temperature.
OBJECTIVE:
- To write a Python script to see the variance of equivalence ratio and adiabatic flame temperature.
- Using the Cantera library to do the same and observe the differences.
- Varying the heat loss factor from 0 to 1 and plotting variation in flame temperature with heat loss.
- To see a variation of adiabatic flame temperature with alkane, alkene, and alkyne.
- To see the effect on AFT by the number of carbon atoms in a chain.
THEORY:
The term "adiabatic flame temperature" (AFT) refers to the greatest temperature that a flame can reach when the environment is adiabatic. Since all combustion reactions will ultimately lose some heat to their environment, this idea is frequently employed in practice as a standard for theoretical performance. However, comprehension of the AFT is crucial for combustion engineers since it sets a maximum temperature that a combustion reaction can achieve and aids in the design and comprehension of combustion systems.
The system's work results in various AFTs for constant volume and constant pressure operations. Because there is no volume change in a constant volume operation, the system does nothing. This indicates that the entire amount of energy generated by the reaction is used to raise the system's temperature, which raises the AFT. As the gas expands, however, in a process with constant pressure, some of the energy produced by the combustion reaction does work on the surroundings. As a result, there is less energy available to raise the temperature, which results in a lower AFT.
We shall derive the equations for the constant pressure and volume cases:
The first law of thermodynamics gives us the following equation:

Since the system is constant volume and consideration is adiabatic the work component and heat component are zero which gives us:

For the constant pressure case, we define displacement work which is:
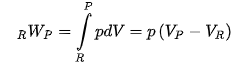
Since the consideration for heat interaction is adiabatic the heat component is the first law equation is zero, which gives us:

The rearranged terms represent the enthalpy of the species, which basically describes the energy of flow systems.

Given that the final enthalpies and moles are often functions of the end temperature, it can be challenging to directly solve this equation for the AFT. However, it is possible to iterate through many potential final temperatures until the aforementioned equation is met using thermodynamic tables or software like Cantera. The AFT is the temperature that fulfills this equation.
Combustion is used to efficiently consume fuel and generate heat. The three elements of combustion are heat, oxygen, and fuel. The most frequent types of fuel used in combustion processes are fossil fuels, which include coal, natural gas, and oil. Hydrocarbons, which are organic molecules made of carbon and hydrogen, are fossil fuels. Consuming (or burning) all of the fuel is referred to as total combustion, and it is essential to do this to enhance combustion efficiency. Methane's reaction is as follows:

The general reaction for any hydrocarbon is:

The equivalence ratio in combustion is a measurement of the difference between the actual fuel-to-oxidizer ratio and the stoichiometric fuel-to-oxidizer ratio. The term stoichiometric ratio describes the ideal ratios of fuel and oxidizer that would permit the reaction to proceed so that all of the fuel is consumed entirely and no oxidizer or fuel is left over.
The combustion equation for lean mixtures (phi<1):

The combustion equation for fuel-rich mixtures (phi>1):

PYTHON AFT CODE:
The code for equivalence ratio and AFT is as follows:
import matplotlib.pyplot as plt
import numpy as np
import math
#calculating the effect of aft on the equivalence ratio
# CH4 + 2(O2 + 3.76N2) = CO2 + 2H2O + 7.52 N2
R = 8.314
def h(T, coeff):
a1 =coeff[0]
a2= coeff [1]
a3= coeff [2]
a4= coeff [3]
a5= coeff [4]
a6 = coeff[5]
return((a1 + (a2*T/2) + (a3*pow(T,2)/3) + (a4*pow(T,3)/4) + (a5*pow(T,4)/5) + a6/T)*R*T)
# low-temperature coefficients
ch4_coeff_l= [5.14987613E+00, -1.36709788E-02, 4.91800599E-05, -4.84743026E-08, 1.66693956E-11, -1.02466476E+04]
o2_coeff_l = [3.78245636E+00, -2.99673416E-03, 9.84730201E-06, -9.68129509E-09, 3.24372837E-12, -1.06394356E+03]
n2_coeff_l = [0.03298677E+02, 0.14082404E-02, -0.03963222E-04, 0.05641515E-07, -0.02444854E-10, -0.10208999E+04]
#high temprature coefficients
n2_coeff_h = [0.02926640E+02, 0.14879768E-02, -0.05684760E-05, 0.10097038E-09, -0.06753351E-13, -0.09227977E+04]
co2_coeff_h =[3.85746029E+00, 4.41437026E-03, -2.21481404E-06, 5.23490188E-10, -4.72084164E-14, -4.87591660E+04]
h2o_coeff_h = [3.03399249E+00, 2.17691804E-03, -1.64072518E-07, -9.70419870E-11, 1.68200992E-14, -3.00042971E+04]
o2_coeff_h = [3.28253784E+00, 1.48308754E-03, -7.57966669E-07, 2.09470555E-10, -2.16717794E-14, -1.08845772E+03]
co_coeff_h = [2.71518561E+00, 2.06252743E-03, -9.98825771E-07, 2.30053008E-10, -2.03647716E-14, -1.41518724E+04]
def f(T,phi): # defining the enthalpies.
if(phi < 1):
h_co2_pr = h(T,co2_coeff_h)
h_h2o_pr = h(T, h2o_coeff_h)
h_n2_pr = h(T, n2_coeff_h)
h_o2_pr = h(T, o2_coeff_h)
h_net_products = h_co2_pr + ((2/phi)-2)*(h_o2_pr)+ (7.52/phi)*(h_n2_pr)+ h_h2o_pr
elif(phi>1):
h_co2_pr = h(T,co2_coeff_h)
h_h2o_pr = h(T, h2o_coeff_h)
h_n2_pr = h(T, n2_coeff_h)
h_o2_pr = h(T, o2_coeff_h)
h_co_pr = h(T, co_coeff_h)
h_net_products = ((4/phi)-3)*(h_co2_pr) + ((4/phi)-4)*h_co_pr + (7.52/phi)*(h_n2_pr)+ 2*(h_h2o_pr)
print(h_net_products)
Tstd = 298.15 # defining the standard temperature
h_ch4_re = h(Tstd, ch4_coeff_l) #defining the enthalpies of reactants
h_o2_re = h(Tstd, o2_coeff_l)
h_n2_re = h(Tstd, n2_coeff_l)
h_net_reactants= h_ch4_re + (2/phi)*(h_o2_re + 3.75*(h_n2_re))
return(h_net_products - h_net_reactants)
# The block of code which does the Newton Rpahson part
def fdash(T, phi):
return ((f(T+1e-6, phi)-f(T, phi))/(1e-6))
T_guess = 1500
tol= 1e-3
phi = np.linspace(0.1, 1.5, 20)
alpha = 0.2
ct = 0
for i in range(0, len(phi)):
while(abs(f(T_guess,phi[i])) > tol):
T_guess= T_guess- alpha*((f(T_guess, phi[i]))/(fdash(T_guess, phi[i])))
print(T_guess)
ct = ct+1
plt.plot(phi[i],T_guess, 'o', color = 'green')
plt.xlabel('Equivalence Ratio')
plt.ylabel('Adiabatic Flame Temprature')
plt.show()
The plot shows the computed AFT as 2810K. The NASA polynomials were used to calculate the enthalpies of the species at the specified temperature, the difference in enthalpies of products and reactants was taken this was defined as a function. The derivative of this function was taken, which was utilized in the Newton-Raphson method to iteratively determine the AFT from a guess temperature.
CANTERA PROGRAM:
The way Cantera computes the AFT is that under the restriction that the system must be in equilibrium, is the temperature at which the system's enthalpy is at its maximum for a particular set of parameters (pressure, composition, etc.). When Cantera uses the 'equilibrate' command, it solves a restricted optimization problem to identify the system's state that maximizes enthalpy while still meeting the equilibrium constraint.
Assuming that each element is conserved, the equilibrium calculation entails identifying the mixture's composition that minimizes the system's Gibbs energy. The procedure presupposes that the reactions advance to the point where their propensities to advance ahead and backward are balanced, and that the mixture's composition stays the same. When internal energy and volume are constant (referred to as "UV").
import matplotlib.pyplot as plt
import cantera as ct
import numpy as np
gas = ct.Solution('gri30.xml')
phi = 0.1
for i in range(0,20):
gas.TPX = 298,101325,{'CH4':1,'O2':2/phi,'N2':7.52/phi}
gas.set_equivalence_ratio(phi,'CH4','O2:1, N2:3.76')
gas.equilibrate('UV','auto')
print(phi, gas.T)
plt.plot(phi,gas.T,'o',color='blue')
phi = phi+0.1
plt.xlabel('Equivalence ratio')
plt.ylabel('Adiabatic Flame Temperature')
plt.show()
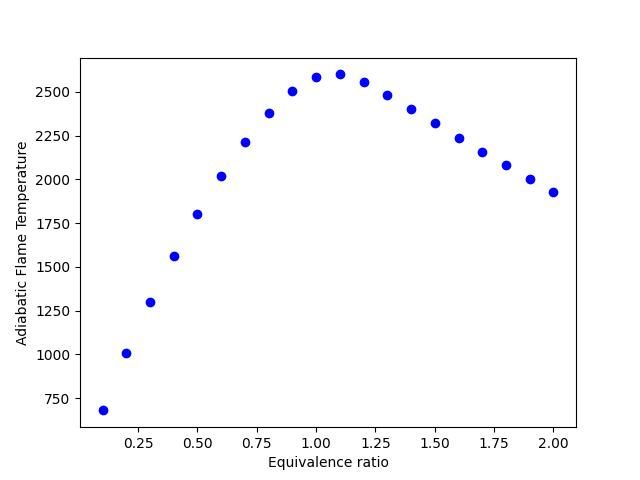
Calculated AFT comes to around 2600K because there are more disassociated products considered here in the Cantera computation in comparison to our previous calculation. This is the screenshot of the data computed.
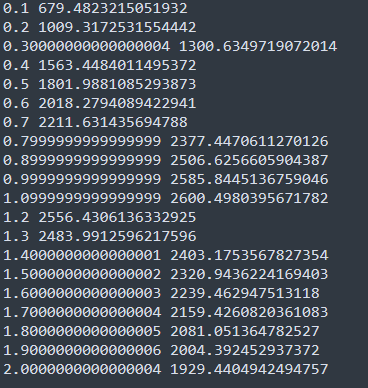
ADIABATIC FLAME TEMPRATURE WITH HEAT LOSS
The main reason for understanding or basically considering heat loss in AFT calculations is to bring a sense of practicality into the analysis. The AFT is calculated under the constraint that the process of combustion is adiabatic, which in practicality is very difficult to achieve.
The flame temperature will be lower than the AFT when heat is transferred from the flame to the environment. The heat produced by this reaction is dispersed among the products (CO2, H2O, and N2) and raises their temperature. The temperature of the products will be lower if some of this heat is lost to the environment since there will be less energy available to heat the products. This heat loss also affects the chemical composition, the amount of disassociated products, and the reaction rates of various intermediate reactions.
The code that includes the effect of heat loss is:
#To calculate the effect of Heat Loss on AFT
#For the Formula
#CH4+2(O2+3.76N2)=CO2+2H2O+7.52N2
import matplotlib.pyplot as plt
import numpy as np
R = 8.314
def h(T,coeffs):
a1 = coeffs[0]
a2 = coeffs[1]
a3 = coeffs[2]
a4 = coeffs[3]
a5 = coeffs[4]
a6 = coeffs[5]
return (a1 + (a2*T/2) + (a3*pow(T,2)/3) + (a4*pow(T,3)/4) + (a5*pow(T,4)/5) + (a6/T))*R*T
# For low-temperature coefficients
CH4_coeffs_l = [5.14987613E+00, -1.36709788E-02, 4.91800599E-05, -4.84743026E-08, 1.66693956E-11, -1.02466476E+04]
O2_coeffs_l = [3.78245636E+00, -2.99673416E-03, 9.84730201E-06, -9.68129509E-09, 3.24372837E-12, -1.06394356E+03]
N2_coeffs_l = [0.03298677E+02, 0.14082404E-02, -0.03963222E-04, 0.05641515E-07, -0.02444854E-10, -0.10208999E+04]
# For high-temperature coefficients
N2_coeffs_h = [0.02926640E+02, 0.14879768E-02, -0.05684760E-05, 0.10097038E-09, -0.06753351E-13, -0.09227977E+04 ]
CO2_coeffs_h = [3.85746029E+00, 4.41437026E-03, -2.21481404E-06, 5.23490188E-10, -4.72084164E-14, -4.87591660E+04]
H20_coeffs_h = [3.03399249E+00,2.17691804E-03,-1.64072518E-07,-9.70419870E-11,1.68200992E-14,-3.00042971E+04]
def f(T,H_loss):
# R in J/mol.k
R = 8.314
T_std = 298.15
LHV = 800950
h_CH4_r = h(T_std,CH4_coeffs_l)
h_O2_r = h(T_std,O2_coeffs_l)
h_N2_r = h(T_std,N2_coeffs_l)
h_CO2_p = h(T,CO2_coeffs_h)
h_N2_p = h(T,N2_coeffs_h)
h_H2O_p = h(T,H20_coeffs_h)
h_reactants = h_CH4_r + 2*h_O2_r + 7.52*h_N2_r
h_products = h_CO2_p + 2*h_H2O_p +7.52*h_N2_p
loss = H_loss*LHV
return h_products - h_reactants + loss
def fprime(T,H_loss):
return (f(T+1e-6,H_loss) - f(T,H_loss))/1e-6
T_guess = 1500
tol = 1e-4
ct = 0
alpha = 1.2
H_loss = np.linspace(0,1,20)
for i in range(0,len(H_loss)):
while ( abs(f( T_guess,H_loss[i] ) ) > tol ):
T_guess = T_guess - alpha * ( f( T_guess,H_loss[i] ) / fprime( T_guess,H_loss[i] ))
ct = ct+1
print(H_loss[i], T_guess)
plt.plot( H_loss[i] ,T_guess, 'o', color='red')
print(ct)
print(T_guess)
plt.xlabel('Heat Loss factor')
plt.ylabel('Flame Temperature(K)')
plt.title('Effect of Heat loss on the Flame Temperature')
plt.show()
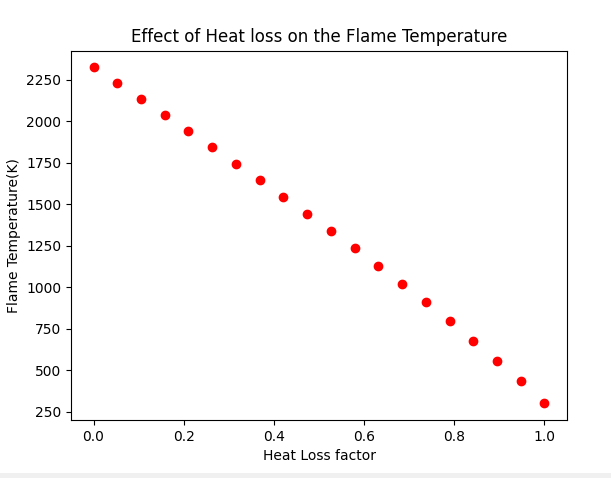
The screenshot of the points, basically the AFT with different loss factors:
From the plot and values we can see that by increasing the loss factor, AFT decreases as most of the heat is lost to the surroundings and less of it contributes to the adiabatic flame temperature.
AFT FOR DIFFERENT HYDROCARBONS (ALKANE, ALKYNE, ALKENE)
The AFT varies with the type of bonds the hydrocarbons share. The bond strength of each hydrocarbon is different and has different energy demands, these energy demands affect the AFT, which we shall see using the previous code.
We shall use the coefficient data for ethane, ethene, and ethyne. We shall define functions for each hydrocarbon group that outputs the enthalpy and the AFT, with a for loop doing the Newton-Rapshon iteration for each hydrocarbon group.
In this code, we have considered 35% heat loss, hence 0.35*LHV
The code is:
#Alkane (ETHANE)
#C2H6 + 3.5O2 +3.5 *3.76 N2 = 2CO2 + 3H2O + 3.5 *3.76 N2 + 0.35* LHV
#Alkene (ETHENE)
#C2H4 + 3O2 + 3 *3.76 N2 = 2CO2 + 2H2O + 3 *3.76 N2 + 0.35* LHV
#Alkyne (ETHYNE)
#C2H2 + 2.5O2 + 2.5 *3.76 N2 = 2CO2 + H2O + 3 *3.76 N2 + 0.35* LHV
import matplotlib.pyplot as plt
import numpy as np
import math
def h(T,coeffs):
R = 8.314
a1=coeffs[0]
a2=coeffs[1]
a3=coeffs[2]
a4=coeffs[3]
a5=coeffs[4]
a6=coeffs[5]
a7=coeffs[6]
return (a1 + (a2*T/2) + (a3*pow(T,2)/3) + (a4*pow(T,3)/4) + (a5*pow(T,4)/5) + (a6/T))*R*T
# For low temprature coefficients
c2h6_coeffs_l =[4.29142492E+00,-5.50154270E-03 ,5.99438288E-05 ,-7.08466285E-08 ,2.68685771E-11,-1.15222055E+04 ,2.66682316E+00]
c2h4_coeffs_l =[3.95920148E+00,-7.57052247E-03 ,5.70990292E-05 ,-6.91588753E-08 ,2.69884373E-11 ,5.08977593E+03 ,4.09733096E+00]
c2h2_coeffs_l =[8.08681094E-01 ,2.33615629E-02,-3.55171815E-05 ,2.80152437E-08,-8.50072974E-12 ,2.64289807E+04 ,1.39397051E+01]
n2_coeffs_l = [0.03298677E+02, 0.14082404E-02,-0.03963222E-04, 0.05641515E-07,-0.02444854E-10,-0.10208999E+04, 0.03950372E+02]
o2_coeffs_l = [3.78245636E+00,-2.99673416E-03, 9.84730201E-06,-9.68129509E-09, 3.24372837E-12,-1.06394356E+03, 3.65767573E+00]
# For high temprature coefficients
n2_coeffs_h = [0.02926640E+02, 0.14879768E-02,-0.05684760E-05, 0.10097038E-09,-0.06753351E-13,-0.09227977E+04, 0.05980528E+02]
co2_coeffs_h = [3.85746029E+00, 4.41437026E-03,-2.21481404E-06, 5.23490188E-10,-4.72084164E-14,-4.87591660E+04, 2.27163806E+00]
h2o_coeffs_h = [3.03399249E+00, 2.17691804E-03,-1.64072518E-07,-9.70419870E-11, 1.68200992E-14,-3.00042971E+04, 4.96677010E+00]
# Alkane
def f_alkane(T):
R = 8.314
T_std = 298.15
LHV = 1428275 #J/mole
h_c2h6_r = h(T_std,c2h6_coeffs_l)
h_o2_r = h(T_std,o2_coeffs_l)
h_n2_r = h(T_std,n2_coeffs_l)
h_co2_p = h(T,co2_coeffs_h)
h_h2o_p = h(T,h2o_coeffs_h)
h_n2_p = h(T,n2_coeffs_h)
H_reactants = h_c2h6_r + 3.5*h_o2_r + (3.5*3.76*h_n2_r)
H_products = 2*h_co2_p+ 3*h_h2o_p + (3.5*3.76*h_n2_p)
return H_products - H_reactants + (0.35*LHV)
def fprime_alkane(T):
return (f_alkane(T+1e-6) - f_alkane(T))/1e-6
# Alkene
def f_alkene(T):
R = 8.314
T_std = 298.15
LHV = 1323474 #J/mole
h_c2h4_r = h(T_std,c2h4_coeffs_l)
h_o2_r = h(T_std,o2_coeffs_l)
h_n2_r = h(T_std,n2_coeffs_l)
h_co2_p = h(T,co2_coeffs_h)
h_h2o_p = h(T,h2o_coeffs_h)
h_n2_p = h(T,n2_coeffs_h)
H_reactants = h_c2h4_r + 3*h_o2_r + (3*3.76*h_n2_r)
H_products = 2*h_co2_p+ 2*h_h2o_p + (3*3.76*h_n2_p)
return H_products - H_reactants + (0.35*LHV)
def fprime_alkene(T):
return (f_alkene(T+1e-6) - f_alkene(T))/1e-6
# THREE : Alkyne
def f_alkyne(T):
R = 8.314
T_std = 298.15
LHV = 1258020 #J/mole
h_c2h2_r = h(T_std,c2h2_coeffs_l)
h_o2_r = h(T_std,o2_coeffs_l)
h_n2_r = h(T_std,n2_coeffs_l)
h_co2_p = h(T,co2_coeffs_h)
h_h2o_p = h(T,h2o_coeffs_h)
h_n2_p = h(T,n2_coeffs_h)
H_reactants = h_c2h2_r + 2.5*h_o2_r + (2.5*3.76*h_n2_r)
H_products = 2*h_co2_p+ h_h2o_p + (3*3.76*h_n2_p)
return H_products - H_reactants + (0.35*LHV)
def fprime_alkyne(T):
return (f_alkyne(T+ 1e-6) - f_alkyne(T))/1e-6
T_alkane_guess = 1500
T_alkene_guess = 1500
T_alkyne_guess = 1500
tol = 1e-4
alpha = 0.8
ct = 0
T_aft = []
#alkane
while(abs(f_alkane(T_alkane_guess)) > tol):
T_alkane_guess = T_alkane_guess - alpha*(f_alkane(T_alkane_guess) / fprime_alkane(T_alkane_guess))
T_aft.append(T_alkane_guess)
#alkene
while(abs(f_alkene(T_alkene_guess)) > tol):
T_alkene_guess = T_alkene_guess - alpha*(f_alkene(T_alkene_guess) / fprime_alkene(T_alkene_guess))
T_aft.append(T_alkene_guess)
#alkyne
while(abs(f_alkyne(T_alkyne_guess)) > tol):
T_alkyne_guess = T_alkyne_guess - alpha*(f_alkyne(T_alkyne_guess) / fprime_alkyne(T_alkyne_guess))
T_aft.append(T_alkyne_guess)
fuel=['Ethane','Ethene','Ethyne']
print(T_aft)
plt.bar(fuel,T_aft)
plt.xlabel('Type of Hydrocarbon')
plt.ylabel(' Flame Temperature(K)')
plt.title('Estimation of FT for 35 Percent of Heat Loss')
plt.show()
As we can see from the plot and the values ethane has the lowest and ethyne has the highest, as more hydrogen bonds have to be broken in the latter, as compared to ethyne the difference in AFT. Also since to break the C-C bond in ethyne more energy is needed hence a greater release of energy.
ADIABATIC FLAME TEMPERATURE WITH THE NUMBER OF CARBON ATOMS
To understand the variation of AFT with the number of carbon atoms we use methane, ethane, and propane.
The code utilized is:
#Code for Methane, Ethene, Ethyne and plot its value against temperature.
#Variation in AFT with change in number of Carbon Atom
#Methane
#CH4 + 2O2 +2 *3.76 N2 = CO2 + 2H2O + 2 *3.76 N2
#Ethane
#C2H6 + 3.5O2 +3.5*3.76 N2 = 2CO2 + 3H2O + 3.5 *3.76 N2
#Propane
#C3H8 + 5O2 + 5*3.76 N2 = 3CO2 + 4H2O + 5*3.76 N2
import matplotlib.pyplot as plt
import numpy as np
import math
R = 8.314
def h(T,co_effs):
a1 = co_effs[0]
a2 = co_effs[1]
a3 = co_effs[2]
a4 = co_effs[3]
a5 = co_effs[4]
a6 = co_effs[5]
a7 = co_effs[6]
return (a1 + (a2*T/2) + (a3*pow(T,2)/3) + (a4*pow(T,3)/4) + (a5*pow(T,4)/5) + (a6/T))*R*T
# For low temprature coefficients
ch4_coeffs_l = [5.14987613E+00,-1.36709788E-02, 4.91800599E-05,-4.84743026E-08, 1.66693956E-11,-1.02466476E+04,-4.64130376E+00]
c2h6_coeffs_l =[4.29142492E+00,-5.50154270E-03, 5.99438288E-05,-7.08466285E-08 ,2.68685771E-11,-1.15222055E+04 ,2.66682316E+00]
c3h8_coeffs_l =[0.93355381E+00, 0.26424579E-01,0.61059727E-05,-0.21977499E-07 ,0.95149253E-11 ,-0.13958520E+05 ,0.19201691E+02]
n2_coeffs_l = [0.03298677E+02, 0.14082404E-02,-0.03963222E-04, 0.05641515E-07,-0.02444854E-10,-0.10208999E+04, 0.03950372E+02]
o2_coeffs_l = [3.78245636E+00,-2.99673416E-03, 9.84730201E-06,-9.68129509E-09, 3.24372837E-12,-1.06394356E+03, 3.65767573E+00]
# For high temprature coefficients
n2_coeffs_h = [0.02926640E+02, 0.14879768E-02,-0.05684760E-05, 0.10097038E-09,-0.06753351E-13,-0.09227977E+04, 0.05980528E+02]
co2_coeffs_h = [3.85746029E+00, 4.41437026E-03,-2.21481404E-06, 5.23490188E-10,-4.72084164E-14,-4.87591660E+04, 2.27163806E+00]
h2o_coeffs_h = [3.03399249E+00, 2.17691804E-03,-1.64072518E-07,-9.70419870E-11, 1.68200992E-14,-3.00042971E+04, 4.96677010E+00]
# For Methane
def f_methane(T):
R = 8.314
T_std = 298.15
LHV = 1428275 #J/mole
h_ch4_r = h(T_std,ch4_coeffs_l)
h_o2_r = h(T_std,o2_coeffs_l)
h_n2_r = h(T_std,n2_coeffs_l)
h_co2_p = h(T,co2_coeffs_h)
h_h2o_p = h(T,h2o_coeffs_h)
h_n2_p = h(T,n2_coeffs_h)
H_reactants = h_ch4_r + 2*h_o2_r + 7.52*h_n2_r
H_products = h_co2_p+ 2*h_h2o_p + 7.52*h_n2_p
return H_products - H_reactants
def fprime_methane(T):
return (f_methane(T+1e-6)-f_methane(T))/1e-6
# For Ethane
def f_ethane(T):
R = 8.314
T_std = 298.15
LHV = 1428275 #J/mole
h_c2h6_r = h(T_std,c2h6_coeffs_l)
h_o2_r = h(T_std,o2_coeffs_l)
h_n2_r = h(T_std,n2_coeffs_l)
h_co2_p = h(T,co2_coeffs_h)
h_h2o_p = h(T,h2o_coeffs_h)
h_n2_p = h(T,n2_coeffs_h)
H_reactants = h_c2h6_r + 3.5*h_o2_r + (3.5*3.76*h_n2_r)
H_products = 2*h_co2_p+ 3*h_h2o_p + (3.5*3.76*h_n2_p)
return H_products - H_reactants
def fprime_ethane(T):
return (f_ethane(T+1e-6)-f_ethane(T))/1e-6
# For Propane
def f_propane(T):
R = 8.314
Tstd = 298.15
LHV = 1428275 #J/mole
h_c3h8_r = h(Tstd,c3h8_coeffs_l)
h_o2_r = h(Tstd,o2_coeffs_l)
h_n2_r = h(Tstd,n2_coeffs_l)
h_co2_p = h(T,co2_coeffs_h)
h_h2o_p = h(T,h2o_coeffs_h)
h_n2_p = h(T,n2_coeffs_h)
H_reactants = h_c3h8_r + 5*h_o2_r + (5*3.76*h_n2_r)
H_products = 3*h_co2_p+ 4*h_h2o_p + (5*3.76*h_n2_p)
return H_products - H_reactants
def fprime_propane(T):
return (f_propane(T+1e-6)-f_propane(T))/1e-6
T_methane_guess = 1500
T_ethane_guess = 1500
T_propane_guess = 1500
tol = 1e-4
alpha = 0.8
ct = 0
T_aft = []
# methane
while(abs(f_methane(T_methane_guess)) > tol):
T_methane_guess = T_methane_guess - alpha*(f_methane(T_methane_guess) / fprime_methane(T_methane_guess))
T_aft.append(T_methane_guess)
# ethane
while(abs(f_ethane(T_ethane_guess)) > tol):
T_ethane_guess = T_ethane_guess - alpha*(f_ethane(T_ethane_guess) / fprime_ethane(T_ethane_guess))
T_aft.append(T_ethane_guess)
# propane
while(abs(f_propane(T_propane_guess)) > tol):
T_propane_guess = T_propane_guess - alpha*(f_propane(T_propane_guess) / fprime_propane(T_propane_guess))
T_aft.append(T_propane_guess)
fuel=['Methane','Ethane','Propane']
print(T_aft)
plt.bar(fuel,T_aft)
plt.xlabel('Type of Hydrocarbon')
plt.ylabel(' Flame Temperature(K)')
plt.title('Effect of alkane carbon atoms on the AFT ')
plt.show()
In general, heavier hydrocarbons like propane (C3H8) tend to have higher adiabatic flame temperatures than lighter hydrocarbons like methane (CH4) or ethane (C2H6). This is primarily because the combustion of heavier hydrocarbons releases more energy (has a higher heat of combustion) due to the greater number of C-H and C-C bonds that are broken and reformed as C=O and H-O bonds, which are more energetically stable.
Furthermore, propane combustion will generally have a higher ratio of CO2 and H2O products, both of which have a higher specific heat capacity than the N2 which makes up the majority of the reactants in air. This means the same amount of heat will cause a smaller increase in temperature in the products, thereby increasing the AFT.